Object Oriented Programming
The procedural approach is the conventional way of writing code in high-level languages where a problem is considered a sequence of things to be performed, such as walking, eating, reading, and so on. A number of functions can be written to accomplish such tasks. The procedural approach organizes a set of computer instructions into groups called procedures - also known as functions.
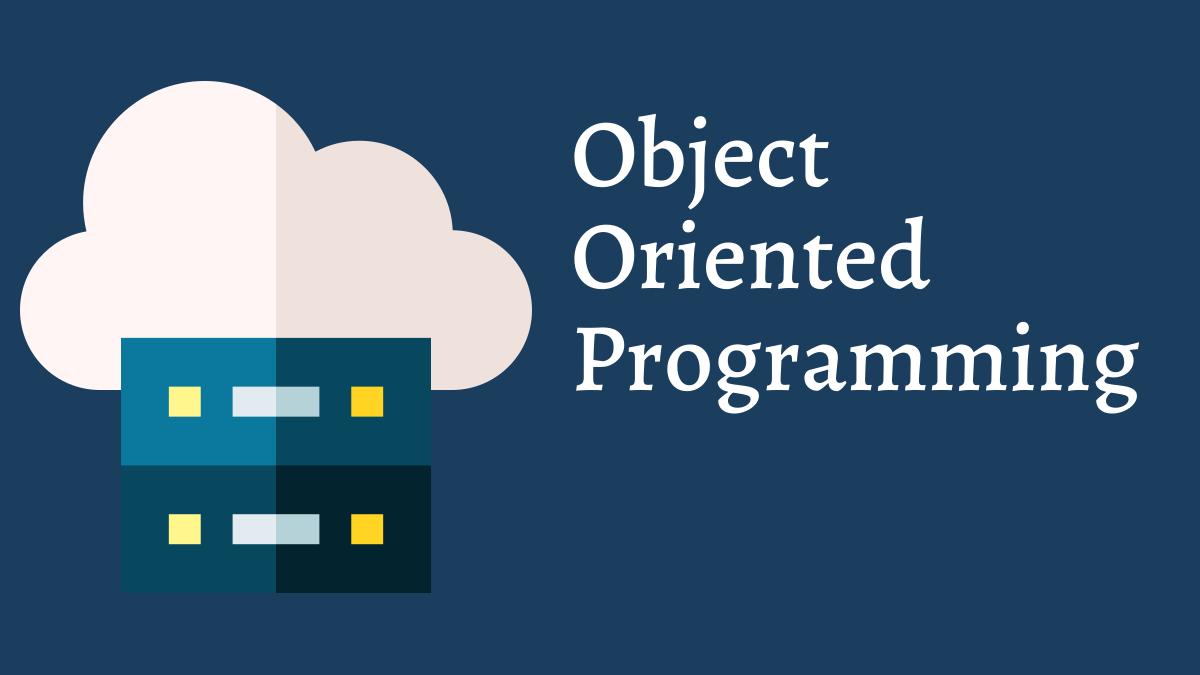
When applications start growing, representing more complex data structures becomes necessary. Primitive types like integers, strings, or arrays are not enough when you want to associate specific behavior to data.
Object oriented programming (OOP) is an approach to programming that uses classes and objects.
Objects and Classes
1. Objects are entities with data and interfaces. They may represent a person, a vehicle, a table fan, or maybe a bank account that plays a role in the program. Data and functions (or methods) live together inside an object.
2. Classes are templates for object creation. Data is the description of an object, while functions are the behaviors of that object, so such definitions of data and methods can be written using a class.
3. Data encapsulation is the wrapping up of data and functions into a single unit - that is, a class. Imagine a capsule with data and functions encapsulated inside so that the outside world cannot access the data as long as we don't expose methods for them. Such insulation of the data from direct access by the program is called data hiding. In short, declaring a class is the encapsulation of data.
4. Data abstraction is the act of representing essential properties and features without giving details. So, the entire entity description remains abstract and the responsibility of detailing the entity can be done via the entity creation process or inheritance.
5. Inheritance is the process of acquiring properties and behaviors of another class so that common properties and behaviors can be reused in a hierarchical manner.
6. Polymorphism is the concept of using the same definition for multiple purposes. For example, flying is a polymorphic behavior, as birds and airplanes have their own different ways of flying.
7. Dynamic binding is the linking of a function call to the code that will be executed in response to the function call. With this concept, the code associated with the given function is unknown until the call is made at runtime. Say that multiple objects implemented the same function differently and at runtime, the code matching the object being referenced would be called.
8. Message passing is the way that objects interact with each other. It involves specifying the object name, the name of the methods, and the information to be sent. For example, if a car is an object, changing speed is a method on it, and speed in kilometres per hour is the speed parameter to be passed. The outside world will use the car object to send the "change speed" message to that parameter.
An object is a complex, user-defined data type. The process of creating an object from a class is called instantiation. An object is an instance of a class.
Properties
Objects have properties, also called attributes. A car may be red, or green - a color property. Properties such as color, size, or model for a car, are stored inside the object. Properties are set up in the class as variables.
The variables that store properties can have default values, can be given values when the object is created, or values can be added or modified later.
Methods
The things objects can do are referred to as responsibilities. For example, a Car object can move forward, stop, back up, and park. Each thing an object can do, each responsibility, is programmed into the class and called a method.
In PHP, methods use the same syntax as functions. Although the code looks like the code for a function, the distinction is that methods are inside a class. It can’t be called independently of an object.
Inheritance
Objects should contain only the properties and methods they need. Child classes inherit all the properties and methods from the parent class. But they can also have their own individual properties.
A child class can contain a method with the same name as a method in a parent class. In that case, the method in the child class takes precedence for a child object.
Developing an Object-Oriented Script
Object-oriented scripts require a lot of planning. You need to plan your objects and their properties and what they can do. Developing object-oriented scripts includes the following procedures:
- Choose the objects
- Choose the properties and methods for each object
- Create the object and put it to work
Creating and Using an Object
After you decide on the design of an object, you can create and then use the object. The steps for creating and using an object are as follows:
1. Write the class statement
The class statement is a PHP statement that is the blueprint for the object. The class statement has a statement block that contains PHP code for all the properties and methods that the object has.
2. Include the class in the script where you want to use the object
You can write the class statement in the script itself. However, it is more common to save the class statement in a separate file and use an include statement to include the class at the beginning of the script that needs to use the object.
3. Create an object in the script
You use a PHP statement to create an object based on the class. This is called instantiation.
4. Use the new object
After you create a new object, you can use it to perform actions. You can use any method that is inside the class statement block.